http://msdn.microsoft.com/en-us/library/bb311042.aspx
Notice:
1.The extension method is defined in another static class other than the "container" class.
2.The extension method should be accessible by the "container" class.
3.When define extension method, the method has first parameter: this "Container" Class Type and "Container" Class variable, and after that, there will follow the other parameters.
4. When use the extension method, the parameters are from the second parameters.
class Program
{
static void Main(string[] args)
{
Cat cat1 = new Cat("Coco", 3);
cat1.Meow(2);
}
}
public class Cat
{
public string Name{get;set;}
public int Age{get;set;}
public Cat(string name, int age) { Name = name; Age = age; }
}
public static class CatExtensions
{
public static void Meow(this Cat cat, int times)
{
for (int i = 0; i < times; i++)
{
Console.WriteLine(cat.Name+": Meow, I am "+cat.Age.ToString()+" years old");
}
}
}
The running result is like:
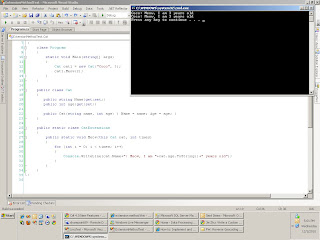
We will find extension method is very useful. I take some another examples,
eg.
public static class IntExtensions
{
public static double Seconds(this int i)
{
return ((double)i /1000);
}
}
when use this we can use 2.Second();
eg.
public static class StringExtensions
{
public static string ReverseString(this string str)
{
.....
}
}
Here is a good reference:
http://msdn.microsoft.com/en-us/vcsharp/bb905825.aspx
it tells how internally extension method works(it is compiled to a static method with ExtensionAttrible), and it also tells how it distinguish from normal instance method by visual studio intelligence.
In Summary, Extension Method is nothing new than the static method. The compiler changed the Extension method into static method when compile the code. But use extension method it make code easier to read, eg. when we use Linq.
No comments:
Post a Comment