Download the unity dll's from http://unity.codeplex.com/
I. Without Registering Type/Instance
By the documents of Unity, we have:
"You can use the Unity container to generate instances of any object that has a public constructor (in other words, objects that you can create using the new operator), without registering a mapping for that type with the container. When you call the Resolve method and specify the default instance of a type that is not registered, the container simply calls the constructor for that type and returns the
result."
We write the following code to test:
class Program
{
static void Main(string[] args)
{
IUnityContainer container = new UnityContainer();
MyService serviceInstance = container.Resolve< MyService>();
serviceInstance.Print("End of 2010.");
}
public class MyService
{
public void Print(string msg)
{
Console.WriteLine("Hello," + msg);
}
}
}
The Unity Application Block exposes two methods for registering types and mappings with the container:
II. Register Type
This method register a type with the container and at the appropriate time the container will build an instance of the type you specified.
Let's first Create an interface called IWriteService,
public interface IWriteService
{
void Write(string msg);
}
And we create 4 concrete classes
public class FirstService : IWriteService
{
public void Write(string msg)
{
Console.WriteLine("First Service: "+msg);
}
}
public class SecondService : IWriteService
{
public void Write(string msg)
{
Console.WriteLine("Second Service: " + msg);
}
}
public class ThirdService : IWriteService
{
public void Write(string msg)
{
Console.WriteLine("Third Service: " + msg);
}
}
public class FourthService : IWriteService
{
public void Write(string msg)
{
Console.WriteLine("Fourth Service: " + msg);
}
}
In the Main entry, we can register the Service with mapping.
IUnityContainer container = new UnityContainer();
//Do not specify the name, will be the default mapping
container.RegisterType< IWriteService, FirstService>();
//notice we use the RegisterType< TFrom, TTo >, this creats a mapping
//we can also use RegisterType< T>
//Specify the name
container.RegisterType< IWriteService, SecondService>("Second");
//We can also use register chain
container.RegisterType< IWriteService, ThirdService>("Third")
.RegisterType< IWriteService, FourthService>("Fourth");
So when resolve, we can specify the name or use the default mapping.
IWriteService serviceInstance1 = container.Resolve< IWriteService>();
serviceInstance1.Write("test");
IWriteService serviceInstance2 = container.Resolve< IWriteService>("Second");
serviceInstance2.Write("test");
IWriteService serviceInstance3 = container.Resolve< IWriteService>("Third");
serviceInstance3.Write("test");
We can also use ResolveAll method to get the IEnumerable< IWriteService> Collection:
IEnumerable< IWriteService> serviceInstances = container.ResolveAll< IWriteService>();
foreach (IWriteService service in serviceInstances)
{
service.Write("good");
}
The source code is at:
https://docs.google.com/uc?id=0BwzR-spEXHBFZjU1ZjUxMWMtNTg1ZS00MWRiLTg2M2MtY2MzZDBiNDUwMTAw&export=download&hl=en
III. Register Instance
We can also register instance to the container.
We write class the following:
public class FifthService : IWriteService
{
public void Write(string msg)
{
Console.WriteLine("Fifth Service: " + msg);
}
}
And in the Main entry, we write code to register and resolve instance like the following:
container.RegisterInstance< IWriteService>(new FifthService());
IWriteService service5 = container.Resolve< IWriteService>();
service5.Write("Register Instance");
Thursday, December 30, 2010
Friday, December 17, 2010
Cast IEnumverable< T> to ObservableCollection< T>
My sample code:
using System.Collections.Generic;
using System.Collections.ObjectModel;
class Program
{
static void Main(string[] args)
{
ObservableCollection< Employee> obc = new ObservableCollection< Employee>(GetEployeeList());
}
public static IEnumerable< Employee> GetEployeeList()
{
for (int i = 0; i < 5; i++)
{
yield return new Employee { ENumber = i + 1, EName = "Name" + (i + 1).ToString() };
}
}
}
public class Employee
{
public int ENumber { get; set; }
public string EName { get; set; }
}
using System.Collections.Generic;
using System.Collections.ObjectModel;
class Program
{
static void Main(string[] args)
{
ObservableCollection< Employee> obc = new ObservableCollection< Employee>(GetEployeeList());
}
public static IEnumerable< Employee> GetEployeeList()
{
for (int i = 0; i < 5; i++)
{
yield return new Employee { ENumber = i + 1, EName = "Name" + (i + 1).ToString() };
}
}
}
public class Employee
{
public int ENumber { get; set; }
public string EName { get; set; }
}
Wednesday, December 15, 2010
About DataContext Class
In Linq to Sql, if we added a Linq to Sql Class, there will created a MyClassDataContext which is inherited from DataContext Class. It is included in System.Data.Linq namespace.
"The DataContext provides connection-like functionality for us and has many capabilities such as executing commands, providing access to collections of entities from the database and identity and tracking services. "
I. Use DataContext to Create a DataBase
We should first create a Table Class included in our DataContext instance, otherwise DB is not allowed to be created.
The Table Attribute comes from using System.Data.Linq.Mapping namespace.
We can use LinqtoSql drag in the desinger to see how a table class looks like, we can check msdn:
http://msdn.microsoft.com/en-us/library/Bb399420(v=VS.90).aspx
Notice, we can use mdf file to create db as well.
Let's take an example:
[Table(Name = "Project")]
public class Project
{
[Column(IsPrimaryKey = true)]
public int PID;
[Column]
public string PName;
[Column]
public string PPerson;
}
And we create a MyDataContext Class overriding the class Context.
public class MyDataContext : DataContext
{
public Table Projects;
public MyDataContext(string constr)
: base(constr)
{
}
}
In this case we can use DataContext to create a database:
MyDataContext mctx = new MyDataContext("server=myServer;Database=MyDB;User Id=Jie Zhu;password=12345");
mctx.CreateDatabase();
2. Use DataContext to Delete a DataBase.
DataContext ctx2 = new DataContext("server=myServer;Database=MyDB;User Id=Jie Zhu;password=12345");
ctx2.DeleteDatabase();
3.Use DataContext to Execute a Sql Command.
DataContext ctx = new DataContext("server=myServer;Database=MyDB;User Id=Jie Zhu;password=12345");
ctx.ExecuteCommand("Insert Project(PID,PName,PPerson) values(1,'Project1','Jack')");
ctx.ExecuteCommand("Insert Project values({0},{1},{2})", 2, "Project2","Amy");
4.Use Context GetTable Method
DataContext ctx = new DataContext("server=myServer;Database=MyDB;User Id=Jie Zhu;password=12345");
foreach (Project p in ctx.GetTable())
{
Console.WriteLine(p.PName);
}
5.Use Context to Translate DataReader
We can use DataContext to translate the DataReader to our objective type.
We create a static method:
static SqlDataReader GetProject(SqlConnection con)
{
con.Open();
SqlDataReader reader;
using (SqlCommand cmd = new SqlCommand("Select * from Project", con))
{
reader = cmd.ExecuteReader();
}
return reader;
}
In this case we can use:
MyDataContext mctx = new MyDataContext("server=myServer;Database=MyDB;User Id=Jie Zhu;password=12345");
foreach (Project p in mctx.Translate(GetProject(new SqlConnection("server=myServer;Database=MyDB;User Id=Jie Zhu;password=12345"))))
{
Console.WriteLine(p.PPerson);
}
6.Use DataContext ExcuteQuery method
IEnumerable ProjectCollection = ctx.ExecuteQuery("Select * from Project");
foreach (Project p in ProjectCollection)
{
Console.WriteLine(p.PID);
}
7. Use DataContext SubmitChanges to Update Table
MyDataContext mctx = new MyDataContext("server=myServer;Database=MyDB;User Id=Jie Zhu;password=12345");
Project p1 = mctx.Projects.Single(p => p.PID == 2);
p1.PPerson = "Erica";
mctx.SubmitChanges();
After we know DataContext clearly here, we know the internal mechanism of Linq to Sql.
For example, we create a Linq to Sql class called MyDataClasses, after database configuration and Table dragging to the designer. It created corresponding DataContext and DataTable classes. You can look at MyDataClasses.designer.cs file.
"The DataContext provides connection-like functionality for us and has many capabilities such as executing commands, providing access to collections of entities from the database and identity and tracking services. "
I. Use DataContext to Create a DataBase
We should first create a Table Class included in our DataContext instance, otherwise DB is not allowed to be created.
The Table Attribute comes from using System.Data.Linq.Mapping namespace.
We can use LinqtoSql drag in the desinger to see how a table class looks like, we can check msdn:
http://msdn.microsoft.com/en-us/library/Bb399420(v=VS.90).aspx
Notice, we can use mdf file to create db as well.
Let's take an example:
[Table(Name = "Project")]
public class Project
{
[Column(IsPrimaryKey = true)]
public int PID;
[Column]
public string PName;
[Column]
public string PPerson;
}
And we create a MyDataContext Class overriding the class Context.
public class MyDataContext : DataContext
{
public Table
public MyDataContext(string constr)
: base(constr)
{
}
}
In this case we can use DataContext to create a database:
MyDataContext mctx = new MyDataContext("server=myServer;Database=MyDB;User Id=Jie Zhu;password=12345");
mctx.CreateDatabase();
2. Use DataContext to Delete a DataBase.
DataContext ctx2 = new DataContext("server=myServer;Database=MyDB;User Id=Jie Zhu;password=12345");
ctx2.DeleteDatabase();
3.Use DataContext to Execute a Sql Command.
DataContext ctx = new DataContext("server=myServer;Database=MyDB;User Id=Jie Zhu;password=12345");
ctx.ExecuteCommand("Insert Project(PID,PName,PPerson) values(1,'Project1','Jack')");
ctx.ExecuteCommand("Insert Project values({0},{1},{2})", 2, "Project2","Amy");
4.Use Context GetTable Method
DataContext ctx = new DataContext("server=myServer;Database=MyDB;User Id=Jie Zhu;password=12345");
foreach (Project p in ctx.GetTable
{
Console.WriteLine(p.PName);
}
5.Use Context to Translate DataReader
We can use DataContext to translate the DataReader to our objective type.
We create a static method:
static SqlDataReader GetProject(SqlConnection con)
{
con.Open();
SqlDataReader reader;
using (SqlCommand cmd = new SqlCommand("Select * from Project", con))
{
reader = cmd.ExecuteReader();
}
return reader;
}
In this case we can use:
MyDataContext mctx = new MyDataContext("server=myServer;Database=MyDB;User Id=Jie Zhu;password=12345");
foreach (Project p in mctx.Translate
{
Console.WriteLine(p.PPerson);
}
6.Use DataContext ExcuteQuery method
IEnumerable
foreach (Project p in ProjectCollection)
{
Console.WriteLine(p.PID);
}
7. Use DataContext SubmitChanges to Update Table
MyDataContext mctx = new MyDataContext("server=myServer;Database=MyDB;User Id=Jie Zhu;password=12345");
Project p1 = mctx.Projects.Single(p => p.PID == 2);
p1.PPerson = "Erica";
mctx.SubmitChanges();
After we know DataContext clearly here, we know the internal mechanism of Linq to Sql.
For example, we create a Linq to Sql class called MyDataClasses, after database configuration and Table dragging to the designer. It created corresponding DataContext and DataTable classes. You can look at MyDataClasses.designer.cs file.
Friday, December 10, 2010
Prevent Sql Injection
What is Sql Injection
Take the page log in as an example:
protected void Button1_Click(object sender, EventArgs e)
{
string cmdstr="Select Count(*) From Users Where Username = '" + UserName.Text + "' And Password = '" + Password.Text + "'";
int result = 0;
using (var conn = new SqlConnection(connect))
{
using (var cmd = new SqlCommand(cmdstr, conn))
{
conn.Open();
result = (int)cmd.ExecuteScalar();
}
}
if (result > 0)
{
Response.Redirect("LoggedIn.aspx");
}
else
{
Label1.Text = "Invalid credentials";
}
}
This kind of code is easy to be get sql injection to be hacked.
For example if a hacker input in the control Username text box the following text:
admin' --
Like the following:

In this case the cmdstr becomes like the following

The hacker bypass the password and use admin account. Here is a link on sql injection cheat sheet:
http://ferruh.mavituna.com/sql-injection-cheatsheet-oku/
In order to prevent Sql Injection, we have 3 method:
1. Use Parameterized Queries
2. Use Stored Procedure
3. Use Linq to Sql
Use Parameterized Queries:
The above code changed to be:
....
string query = "Select * From User Where UserName= @UserName and Password=@PassWord";
using (var conn = new SqlConnection(connect))
{
using (var cmd = new SqlCommand(query, conn))
{
cmd.Parameters.Add("@UserName", SqlDbType.VarChar);
cmd.Parameters["@UserName"].Value = UserName.Text.ToString();
cmd.Parameters.Add("@PassWord", SqlDbType.VarChar);
cmd.Parameters["@PassWord"].Value = PassWord.Text.ToString();
conn.Open()
...
For the Stored Procedure which as parameters, it the similar.
For the Linq to Sql, it need another topic.
Take the page log in as an example:
protected void Button1_Click(object sender, EventArgs e)
{
string cmdstr="Select Count(*) From Users Where Username = '" + UserName.Text + "' And Password = '" + Password.Text + "'";
int result = 0;
using (var conn = new SqlConnection(connect))
{
using (var cmd = new SqlCommand(cmdstr, conn))
{
conn.Open();
result = (int)cmd.ExecuteScalar();
}
}
if (result > 0)
{
Response.Redirect("LoggedIn.aspx");
}
else
{
Label1.Text = "Invalid credentials";
}
}
This kind of code is easy to be get sql injection to be hacked.
For example if a hacker input in the control Username text box the following text:
admin' --
Like the following:

In this case the cmdstr becomes like the following

The hacker bypass the password and use admin account. Here is a link on sql injection cheat sheet:
http://ferruh.mavituna.com/sql-injection-cheatsheet-oku/
In order to prevent Sql Injection, we have 3 method:
1. Use Parameterized Queries
2. Use Stored Procedure
3. Use Linq to Sql
Use Parameterized Queries:
The above code changed to be:
....
string query = "Select * From User Where UserName= @UserName and Password=@PassWord";
using (var conn = new SqlConnection(connect))
{
using (var cmd = new SqlCommand(query, conn))
{
cmd.Parameters.Add("@UserName", SqlDbType.VarChar);
cmd.Parameters["@UserName"].Value = UserName.Text.ToString();
cmd.Parameters.Add("@PassWord", SqlDbType.VarChar);
cmd.Parameters["@PassWord"].Value = PassWord.Text.ToString();
conn.Open()
...
For the Stored Procedure which as parameters, it the similar.
For the Linq to Sql, it need another topic.
Tuesday, December 7, 2010
Difference between override and new
the keyword "new" doesn't change the base class method, while the keyword "override" changes.
We have these code:
class Program
{
static void Main(string[] args)
{
DerivedClass dc = new DerivedClass();
BaseClass bc = new DerivedClass() as BaseClass;
dc.Print();
bc.Print();
}
}
public abstract class BaseClass
{
public virtual void Print()
{
Console.WriteLine("This is base class");
}
}
public class DerivedClass : BaseClass
{
public new void Print()
{
Console.WriteLine("This is derived class");
}
}
The running results are:
This is derived class
This is base class
while if we wrote code below:
class Program
{
static void Main(string[] args)
{
DerivedClass dc = new DerivedClass();
BaseClass bc = new DerivedClass() as BaseClass;
dc.Print();
bc.Print();
}
}
public abstract class BaseClass
{
public virtual void Print()
{
Console.WriteLine("This is base class");
}
}
public class DerivedClass : BaseClass
{
public override void Print()
{
Console.WriteLine("This is derived class");
}
}
The running results are:
This is derived class
This is derived class
We have these code:
class Program
{
static void Main(string[] args)
{
DerivedClass dc = new DerivedClass();
BaseClass bc = new DerivedClass() as BaseClass;
dc.Print();
bc.Print();
}
}
public abstract class BaseClass
{
public virtual void Print()
{
Console.WriteLine("This is base class");
}
}
public class DerivedClass : BaseClass
{
public new void Print()
{
Console.WriteLine("This is derived class");
}
}
The running results are:
This is derived class
This is base class
while if we wrote code below:
class Program
{
static void Main(string[] args)
{
DerivedClass dc = new DerivedClass();
BaseClass bc = new DerivedClass() as BaseClass;
dc.Print();
bc.Print();
}
}
public abstract class BaseClass
{
public virtual void Print()
{
Console.WriteLine("This is base class");
}
}
public class DerivedClass : BaseClass
{
public override void Print()
{
Console.WriteLine("This is derived class");
}
}
The running results are:
This is derived class
This is derived class
Thursday, December 2, 2010
C# 4.0 features -- dynamic typing(1)
For this article, I am going to write what I understand so far. When I have more knowledge on dynamic, I will write more.
We write a method:
public static int GetLength(dynamic myvar)
{
return myvar.Length;
}
The dynamic keyword represents an object which will be resolved in runtime.
so in the Main entry, if we have an string object, it has Length property, we can use:
string mystring="this is for test";
int i=GetLength(mystring);
And also for an array, string[] myarr=new string[3]{"Test","Dynamic","Typing"};
array also have:
int j=GetLength(myarr);
There is no compile time error and there is no run time error.
But if we use int, it doesn't have property Length; int a=5;
int k=GetLength(a);
There is no compile time error. But in the run time, the dynamic object is resolved to int variable, and it doesn't have Length property, there will come out exception.
I wrote the following test code:
public class MyClass1
{
public int Length;
}
public class MyClass2
{ }
class Program
{
static void Main(string[] args)
{
MyClass1 myClass1=new MyClass1(){Length=3};
Console.WriteLine(GetLength(myClass1));
MyClass2 myClass2 = new MyClass2();
Console.WriteLine(GetLength(myClass2));
}
public static int GetLength(dynamic myvar)
{
return myvar.Length;
}
}
The above code explain the dynamic object, the dynamic objects are resolved to myClass1 and myClass2 respectively in the run time. In the compile time, the is no problem at all, but as myClass2 doesn't have Length property, there will have run time exception for the part: GetLength(myClass2). It will say, "RuntimeBinder exception is unhandled" as below:

The difference between "dynamic" and "var" keywords:
var is type inference, the type is determined at compile time, that means the type is determined when we assign the value to var variable.
dynamic the type is resolved at runtime, that is the dynamic type will be replaced by the actual type in run time.
Why dynamic?
To my understanding, C# is a static programming language, it do type check at compile time to improve the performance. But dynamic language such as Python which determine the type at run time. .Net 4.0 has the dynamic language runtime(DLR) which let the C# code by pass compile time type checking. So DLR can be use to programming interop with the dynamic language.
It is useful when we don't know the types in some object such as COM objects and it use IDispatch doing late binding. So C# programming in COM objects which do late binding will also have interop, and we may need to use dynamic.
Developers may use System.Reflection to do the late binding, and dynamic will from programming language to allow late binding.
And when people work on XML/HTML DOM objects, when we don't know some object types, it is needed to use dynamic.
We write a method:
public static int GetLength(dynamic myvar)
{
return myvar.Length;
}
The dynamic keyword represents an object which will be resolved in runtime.
so in the Main entry, if we have an string object, it has Length property, we can use:
string mystring="this is for test";
int i=GetLength(mystring);
And also for an array, string[] myarr=new string[3]{"Test","Dynamic","Typing"};
array also have:
int j=GetLength(myarr);
There is no compile time error and there is no run time error.
But if we use int, it doesn't have property Length; int a=5;
int k=GetLength(a);
There is no compile time error. But in the run time, the dynamic object is resolved to int variable, and it doesn't have Length property, there will come out exception.
I wrote the following test code:
public class MyClass1
{
public int Length;
}
public class MyClass2
{ }
class Program
{
static void Main(string[] args)
{
MyClass1 myClass1=new MyClass1(){Length=3};
Console.WriteLine(GetLength(myClass1));
MyClass2 myClass2 = new MyClass2();
Console.WriteLine(GetLength(myClass2));
}
public static int GetLength(dynamic myvar)
{
return myvar.Length;
}
}
The above code explain the dynamic object, the dynamic objects are resolved to myClass1 and myClass2 respectively in the run time. In the compile time, the is no problem at all, but as myClass2 doesn't have Length property, there will have run time exception for the part: GetLength(myClass2). It will say, "RuntimeBinder exception is unhandled" as below:

The difference between "dynamic" and "var" keywords:
var is type inference, the type is determined at compile time, that means the type is determined when we assign the value to var variable.
dynamic the type is resolved at runtime, that is the dynamic type will be replaced by the actual type in run time.
Why dynamic?
To my understanding, C# is a static programming language, it do type check at compile time to improve the performance. But dynamic language such as Python which determine the type at run time. .Net 4.0 has the dynamic language runtime(DLR) which let the C# code by pass compile time type checking. So DLR can be use to programming interop with the dynamic language.
It is useful when we don't know the types in some object such as COM objects and it use IDispatch doing late binding. So C# programming in COM objects which do late binding will also have interop, and we may need to use dynamic.
Developers may use System.Reflection to do the late binding, and dynamic will from programming language to allow late binding.
And when people work on XML/HTML DOM objects, when we don't know some object types, it is needed to use dynamic.
Wednesday, December 1, 2010
Write a Custom Extension Method
Let's follow this tutorial to write a custom Extension method:
http://msdn.microsoft.com/en-us/library/bb311042.aspx
Notice:
1.The extension method is defined in another static class other than the "container" class.
2.The extension method should be accessible by the "container" class.
3.When define extension method, the method has first parameter: this "Container" Class Type and "Container" Class variable, and after that, there will follow the other parameters.
4. When use the extension method, the parameters are from the second parameters.
class Program
{
static void Main(string[] args)
{
Cat cat1 = new Cat("Coco", 3);
cat1.Meow(2);
}
}
public class Cat
{
public string Name{get;set;}
public int Age{get;set;}
public Cat(string name, int age) { Name = name; Age = age; }
}
public static class CatExtensions
{
public static void Meow(this Cat cat, int times)
{
for (int i = 0; i < times; i++)
{
Console.WriteLine(cat.Name+": Meow, I am "+cat.Age.ToString()+" years old");
}
}
}
The running result is like:
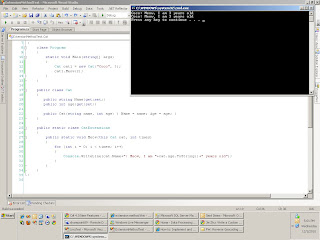
We will find extension method is very useful. I take some another examples,
eg.
public static class IntExtensions
{
public static double Seconds(this int i)
{
return ((double)i /1000);
}
}
when use this we can use 2.Second();
eg.
public static class StringExtensions
{
public static string ReverseString(this string str)
{
.....
}
}
Here is a good reference:
http://msdn.microsoft.com/en-us/vcsharp/bb905825.aspx
it tells how internally extension method works(it is compiled to a static method with ExtensionAttrible), and it also tells how it distinguish from normal instance method by visual studio intelligence.
In Summary, Extension Method is nothing new than the static method. The compiler changed the Extension method into static method when compile the code. But use extension method it make code easier to read, eg. when we use Linq.
http://msdn.microsoft.com/en-us/library/bb311042.aspx
Notice:
1.The extension method is defined in another static class other than the "container" class.
2.The extension method should be accessible by the "container" class.
3.When define extension method, the method has first parameter: this "Container" Class Type and "Container" Class variable, and after that, there will follow the other parameters.
4. When use the extension method, the parameters are from the second parameters.
class Program
{
static void Main(string[] args)
{
Cat cat1 = new Cat("Coco", 3);
cat1.Meow(2);
}
}
public class Cat
{
public string Name{get;set;}
public int Age{get;set;}
public Cat(string name, int age) { Name = name; Age = age; }
}
public static class CatExtensions
{
public static void Meow(this Cat cat, int times)
{
for (int i = 0; i < times; i++)
{
Console.WriteLine(cat.Name+": Meow, I am "+cat.Age.ToString()+" years old");
}
}
}
The running result is like:
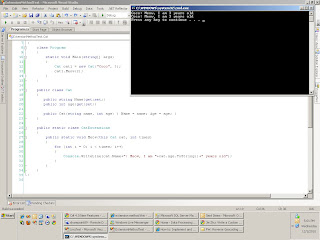
We will find extension method is very useful. I take some another examples,
eg.
public static class IntExtensions
{
public static double Seconds(this int i)
{
return ((double)i /1000);
}
}
when use this we can use 2.Second();
eg.
public static class StringExtensions
{
public static string ReverseString(this string str)
{
.....
}
}
Here is a good reference:
http://msdn.microsoft.com/en-us/vcsharp/bb905825.aspx
it tells how internally extension method works(it is compiled to a static method with ExtensionAttrible), and it also tells how it distinguish from normal instance method by visual studio intelligence.
In Summary, Extension Method is nothing new than the static method. The compiler changed the Extension method into static method when compile the code. But use extension method it make code easier to read, eg. when we use Linq.
Use Linq Query and Extension Method two styles to get IEnumerable Collections
for example there is a IEnumerable Object, we take an Array as an example:
string [5] arr=new string[5]{"bolt","create","eat","bat","boat"};
Use normal Linq query, that is
var q=from str in arr
where str.StartWith("b")
order by str.Length
select str;
Here we get an IEnumerable q;
We can also use Extension method for the class which implements IEnumerable interface.
var q=arr.Where(str=>str.StartWith("b"))
.OrderBy(str=>str.Length);
Notice the extension method Where(), OrderBy(), the parameter should be an predefined delegate --Predicate to call a function with boolean returned value.
As we know, Predicate, Action, Func can all be written in lambda expression.
So use the extension method, the format should be like: arr.Where(str=>str.StartWith("b")).
Let's go to definition of Where extension method:
public static IEnumerable Where(this IEnumerable source, Func predicate);
string [5] arr=new string[5]{"bolt","create","eat","bat","boat"};
Use normal Linq query, that is
var q=from str in arr
where str.StartWith("b")
order by str.Length
select str;
Here we get an IEnumerable q;
We can also use Extension method for the class which implements IEnumerable interface.
var q=arr.Where(str=>str.StartWith("b"))
.OrderBy(str=>str.Length);
Notice the extension method Where(), OrderBy(), the parameter should be an predefined delegate --Predicate to call a function with boolean returned value.
As we know, Predicate, Action, Func can all be written in lambda expression.
So use the extension method, the format should be like: arr.Where(str=>str.StartWith("b")).
Let's go to definition of Where extension method:
public static IEnumerable
Anonymous Class if the variable order not the same, the class types are not the same
I do some test with Anonymous Class:
var s1 = new { Name = "Tom", age = 35 };
var s2 = new { Name = "Eric", age = 35 };
var s3 = new { Name = "Eric", age = 35 };
var s4 = new { age = 35, Name = "Eric" };
Debug.Assert(s1 == s2);
//s1==s2 returns false
Debug.Assert(s1.age == s2.age);
//s1.age == s2.age retuns true
Debug.Assert(s2 == s3);
//s2==s3 returns false
Debug.Assert(s3== s4);
//There will have compile error in this case, as s3, s4 the
//anonymous class, the member orders are differenct, s3,s4 are
//considered as different types, compiling error out
Debug.Assert(s2.GetType() == s2.GetType());
//s2.GetType() == s3.GetType() retuns true
Debug.Assert(s3.GetType() == s4.GetType());
//s3.GetType() == s4.GetType() returns false
var s1 = new { Name = "Tom", age = 35 };
var s2 = new { Name = "Eric", age = 35 };
var s3 = new { Name = "Eric", age = 35 };
var s4 = new { age = 35, Name = "Eric" };
Debug.Assert(s1 == s2);
//s1==s2 returns false
Debug.Assert(s1.age == s2.age);
//s1.age == s2.age retuns true
Debug.Assert(s2 == s3);
//s2==s3 returns false
Debug.Assert(s3== s4);
//There will have compile error in this case, as s3, s4 the
//anonymous class, the member orders are differenct, s3,s4 are
//considered as different types, compiling error out
Debug.Assert(s2.GetType() == s2.GetType());
//s2.GetType() == s3.GetType() retuns true
Debug.Assert(s3.GetType() == s4.GetType());
//s3.GetType() == s4.GetType() returns false
Subscribe to:
Posts (Atom)